Java xml 파일 생성 및 내용 출력 방법 jaxb marshalling
자바 클래스를 xml 로 변환하는 방법은 아래의 Link를 참고하세요.
Link : JAVA Class -> JSON, XML 변환 출력 jaxb marshalling 마샬링 작스비
JAVA Class -> JSON, XML 변환 출력 jaxb marshalling 마샬링 작스비
전자정부 OpenAPI JAVA Class -> JSON XML 변환 출력 jaxb marshalling 마샬링 작스비 이번 포스팅에서는 전자정부프레임워크와 Spring-oxm, jaxb, marshalling 을 활용해 VO Class를 json과 xml로 변환해 출력.....
aljjabaegi.tistory.com
이번 포스팅에서는 간단하게 vo class를 만들어 xml 로 변환하고
xml 파일을 만들어 파일 내의 내용을 출력하는 방법을 알아보도록 하겠습니다.
우선 필요한 라이브러리는 3개.
import javax.xml.bind.JAXBContext;
import javax.xml.bind.JAXBException;
import javax.xml.bind.Marshaller;
자동으로 추가가되지 않는다면 Link를 참고해 dependency를 추가해주세요!
JAXBContext jaxc = null;
Marshaller marshaller = null;
File testFile = null;
TestVO test = new TestVO();
try {
jaxc = JAXBContext.newInstance(TestVO.class);
} catch (JAXBException e) {
LOGGER.error("Jaxb instance 생성 에러", e);
}
xml 구조체를 생성할 최상위 객체(TestVO) 로 새로운 인스턴스를 생성합니다.
xml 파일을 만들것이기 때문에 파일객체도 초기화 해 둡니다.
TestVO는 아래와 같습니다.
import javax.xml.bind.annotation.XmlAccessType;
import javax.xml.bind.annotation.XmlAccessorType;
import javax.xml.bind.annotation.XmlAttribute;
import javax.xml.bind.annotation.XmlElement;
import javax.xml.bind.annotation.XmlRootElement;
import org.springframework.stereotype.Component;
@XmlRootElement(name = "Test")
@XmlAccessorType(XmlAccessType.FIELD)
@Component
public class TestVO {
@XmlAttribute
private String version;
@XmlAttribute
private String name;
@XmlElement(name="value")
private TestValueVO testValue;
public String getVersion() {
return version;
}
public void setVersion(String version) {
this.version = version;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public TestValueVO getTestValue() {
return testValue;
}
public void setTestValue(TestValueVO testValue) {
this.testValue = testValue;
}
}
TestValueVO는 아래와 같습니다.
import javax.xml.bind.annotation.XmlAccessType;
import javax.xml.bind.annotation.XmlAccessorType;
import javax.xml.bind.annotation.XmlElement;
import javax.xml.bind.annotation.XmlRootElement;
import org.springframework.stereotype.Component;
@XmlRootElement(name = "value")
@XmlAccessorType(XmlAccessType.FIELD)
@Component
public class TestValueVO {
@XmlElement(name="GPS_X")
private String gpsX;
@XmlElement(name="GPS_Y")
private String gpsY;
@XmlElement(name="ADDRESS")
private String addr;
public String getGpsX() {
return gpsX;
}
public void setGpsX(String gpsX) {
this.gpsX = gpsX;
}
public String getGpsY() {
return gpsY;
}
public void setGpsY(String gpsY) {
this.gpsY = gpsY;
}
public String getAddr() {
return addr;
}
public void setAddr(String addr) {
this.addr = addr;
}
}
이제 각 데이터에 값을 넣고 File도 생성합니다.
test.setName("xmlTest");
test.setVersion("1.0");
TestValueVO testValue = new TestValueVO();
testValue.setGpsX("37.401675");
testValue.setGpsY("126.961761");
testValue.setAddr("경기도 안양시 동안구 관양2동 743");
test.setTestValue(testValue);
testFile = new File("request.xml");
이제 마샬러의 프로퍼티를 설정하고 변환할 객체와 객체를 output 할 file을 매개변수로
marshal 메서드를 실행합니다.
프로퍼티에 대한 설명은 아래의 Link를 참고하세요.
Link : https://docs.oracle.com/javase/7/docs/api/javax/xml/bind/Marshaller.html
Marshaller (Java Platform SE 7 )
Allow an application to register a validation event handler. The validation event handler will be called by the JAXB Provider if any validation errors are encountered during calls to any of the marshal API's. If the client application does not register a v...
docs.oracle.com
try {
marshaller = jaxc.createMarshaller();
marshaller.setProperty(Marshaller.JAXB_ENCODING, "utf-8");
marshaller.setProperty(Marshaller.JAXB_FORMATTED_OUTPUT, Boolean.TRUE);
marshaller.marshal( test, testFile );
} catch (JAXBException e) {
e.printStackTrace();
}
이제 만들어진 객체가 생성된 파일에 xml 의 형태로 입력이 됩니다.
xml 파일이 생성이 된 것이죠.
데이터가 제대로 들어갔는지 파일 내 데이터를 확인해 보도록 하겠습니다.
try{
FileReader filereader = new FileReader(testFile);
int singleCh = 0;
while((singleCh = filereader.read()) != -1){
System.out.print((char)singleCh);
}
filereader.close();
}catch(Exception e){
LOGGER.error("file read error");
}
[출력결과]
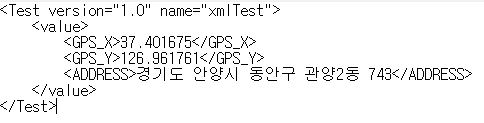
위와 같이 VO 클래스에 set 한 데이터가 xml 형태로 출력이 되는 것을 확인 하실 수 있습니다.
'Programing > JAVA' 카테고리의 다른 글
Java 클래스 변수명과 값 출력하기 how to get name and value of class (0) | 2019.09.02 |
---|---|
Java String 에 대해 깊게 파고들어 보자~! (1) | 2019.08.01 |
Java 자바 UNIX Timestamp 변환 timestamp to date String (0) | 2019.07.17 |
Java Exception 처리 예외 처리 try catch 알짜만 빼먹기 (1) | 2019.07.11 |
[launch4j] jar파일로 exe 파일만드는 방법 how to make exe file to jar file (1) | 2019.06.11 |
댓글